Table Of Content
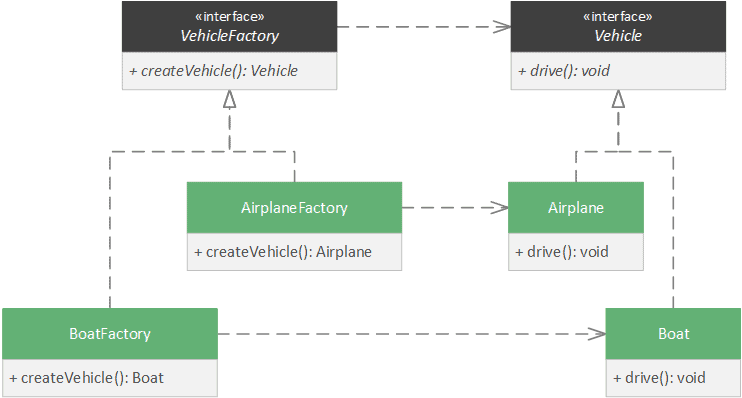
The Factory Design Pattern falls under the Creational Design Patterns Category. As part of this article, we will discuss the following pointers related to the Factory Design Pattern. The difference is in the interface that exposes to support creating any type of object. The builder parameter can be any object that implements the callable interface. This means a Builder can be a function, a class, or an object that implements .__call__(). This is important because if it doesn’t, then the client will have to complete the initialization and use complex conditional code to fully initialize the provided objects.
History of patterns
This is the interface or abstract class for the objects that the factory method creates. The Product defines the common interface for all objects that the factory method can create. We don't want to use reflection but in the same time we want to have a reduced coupling between the factory and concrete products. Since the factory should be unaware of products we have to move the creation of objects outside of the factory to an object aware of the concrete products classes. As we saw in the previous paragraph the factory object uses internally a HashMap to keep the mapping between parameters (in our case Strings) and concrete products class. The registration is made from outside of the factory and because the objects are created using reflection the factory is not aware of the objects types.
Concrete Creator
But now you need to tell the main UIFramework class to use the new button subclass instead of a default one. The Factory Method separates product construction code from the code that actually uses the product. Therefore it’s easier to extend the product construction code independently from the rest of the code. Use Simple Factory Pattern when you don’t know which concrete type you should work with.
Below is the complete combined code for the Factory Method Pattern in C++:
The best approach is to define a new type of object that provides a general interface and is responsible for the creation of a concrete service. The Builder object has all the logic to create and initialize a service instance. You will implement a Builder object for each of the supported services. Ideally, the design should support adding serialization for new objects by implementing new classes without requiring changes to the existing implementation.
Adoption of Cloud Native Architecture, Part 2: Stabilization Gaps and Anti-Patterns - InfoQ.com
Adoption of Cloud Native Architecture, Part 2: Stabilization Gaps and Anti-Patterns.
Posted: Wed, 27 May 2020 07:00:00 GMT [source]
However, you want to decouple the client code from the concrete product creation logic to enhance flexibility and maintainability. Additionally, you want to allow for easy extension by adding new product types in the future without modifying existing code. The code that uses the factory method (often called the client code) doesn’t see a difference between the actual products returned by various subclasses. The client knows that all transport objects are supposed to have the deliver method, but exactly how it works isn’t important to the client. In this client code, we first create instances of the concrete creators (circleFactory and squareFactory) and then use them to create instances of concrete products (cirlce and square).
Factory Method in C++ / Design Patterns
They all define a client that depends on a common interface known as the product. They all provide a means to identify the concrete implementation of the product, so they all can use Factory Method in their design. Factory Method should be used in every situation where an application (client) depends on an interface (product) to perform a task and there are multiple concrete implementations of that interface.
So, create a class file named CreditCard.cs and copy and paste the following code. As you can see, we created the CreditCard interface with three methods per our requirements. Notice that SpotifyServiceBuilder keeps the service instance around and only creates a new one the first time the service is requested. This avoids going through the authorization process multiple times as specified in the requirements.
Each concrete product (Circle and Square) provides its implementation of the draw() method. This is an abstract class or an interface that declares the factory method. The creator typically contains a method that serves as a factory for creating objects. It may also contain other methods that work with the created objects. Please note the procedural switch-case (noob) implementation is the simplest, violates the OCP principle is used only to explain the theory. The only wise way to use it is for temporary modules until it is replaced with a real factory.
Factory method design pattern in Java
The example above shows that, to access a music service, music.factory.create() is called. Other developers might believe that a new instance is created every time and decide that they should keep around the service instance to avoid the slow initialization process. A general purpose Object Factory (ObjectFactory) can leverage the generic Builder interface to create all kinds of objects.
Their purpose is to make the process of creating objects simpler, more modular, and more scalable. Design patterns are a collection of programming methodologies used in day-to-day programming. They represent solutions to some commonly occurring problems in the programming industry, which have intuitive solutions.
The format is used to identify the concrete implementation of the Serializer and is resolved by the factory object. The serializable parameter refers to another abstract interface that should be implemented on any object type you want to serialize. For each variant, we have a concrete factory class like RetroFurnitureFactory.
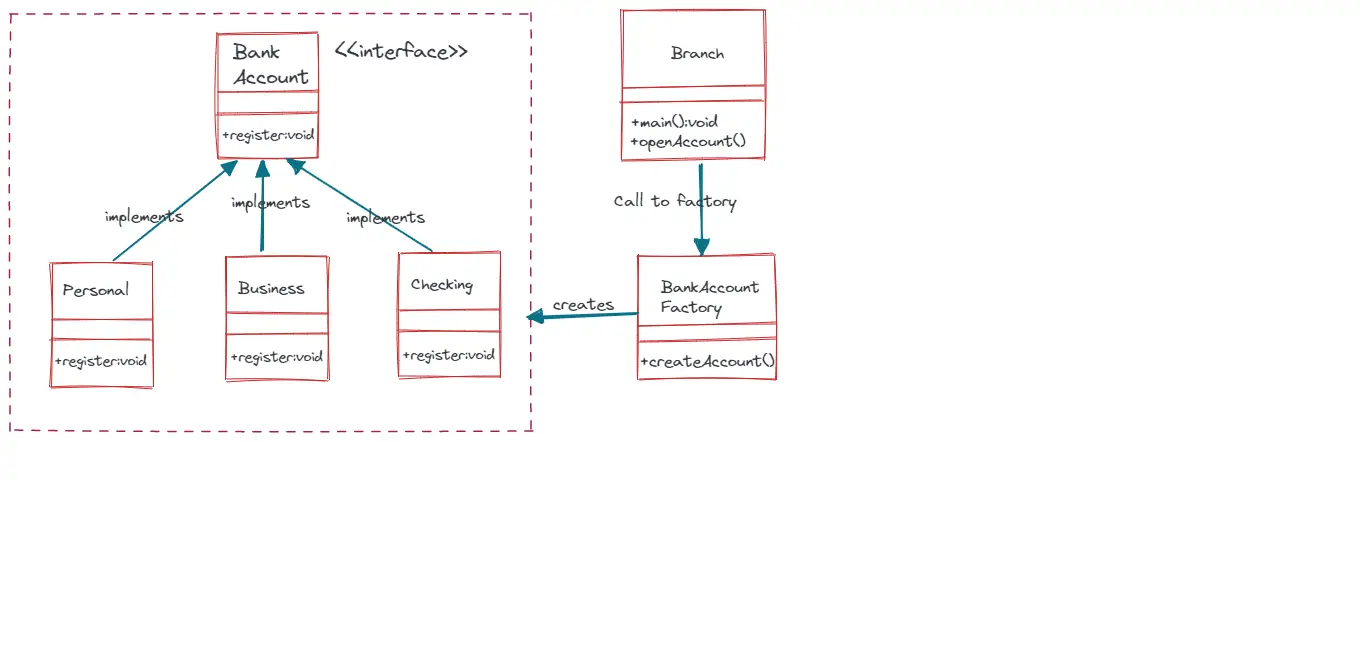
To solve the problem mentioned above, we can apply the Factory Design Pattern. Creator will only depend on abstract product type without knowing which concrete type it’s dealing with. Through this article, I have listed what a Factory Design Pattern is and how to implement a Factory. I hope to receive contributions from everyone so that the programming community can continue to develop, and especially so that future articles can be better. This goes on as you add more related variations of a logical collection - all spaceships for instance.
No comments:
Post a Comment